Generate PDF Dynamically
This page shows you how to generate a PDF from input data, preview it in a Document Viewer widget, and send it as an email attachment.
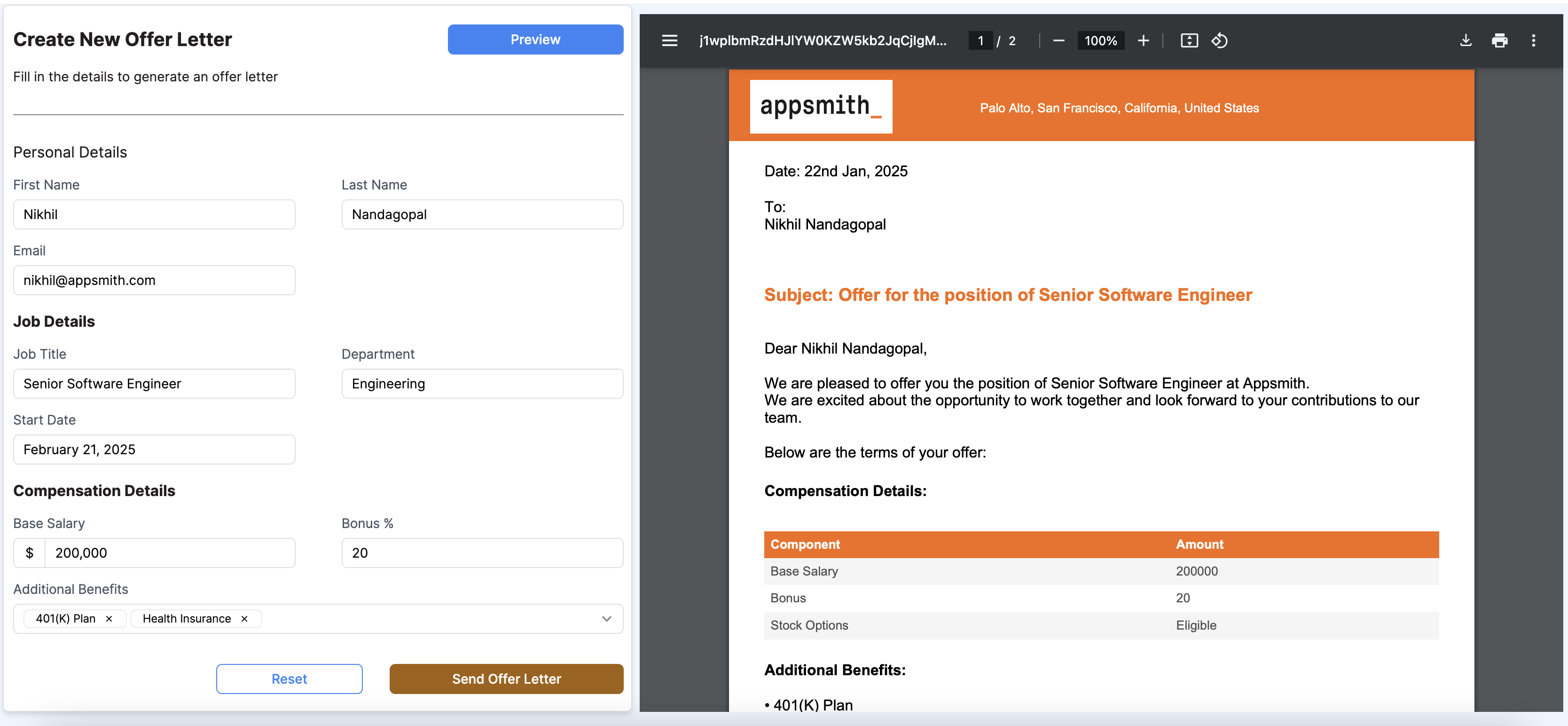
- On the canvas, add the necessary widgets, such as Text Input, Dropdown, and Date Picker, to capture the information needed to generate the PDF.
Example: If you want to generate an offer letter, add widgets to capture details such as the recipient's name, job title, start date, salary, and benefits, which are included in the offer letter PDF.
- Open the Libraries section from the left pane, click the + icon, and add a JavaScript library to generate the PDF.
Ensure the library is available in ECMAScript Modules (ESM) or Universal Module Definition (UMD) format from a reliable CDN, such as jsDelivr or UNPKG.
Example:
- To generate a PDF, import the jsPDF library:
https://unpkg.com/jspdf@latest/dist/jspdf.umd.min.js
- To enhance the PDF generation with tables and other UI components, import the jsPDF AutoTable library:
https://unpkg.com/jspdf-autotable@latest/dist/jspdf.plugin.autotable.min.js
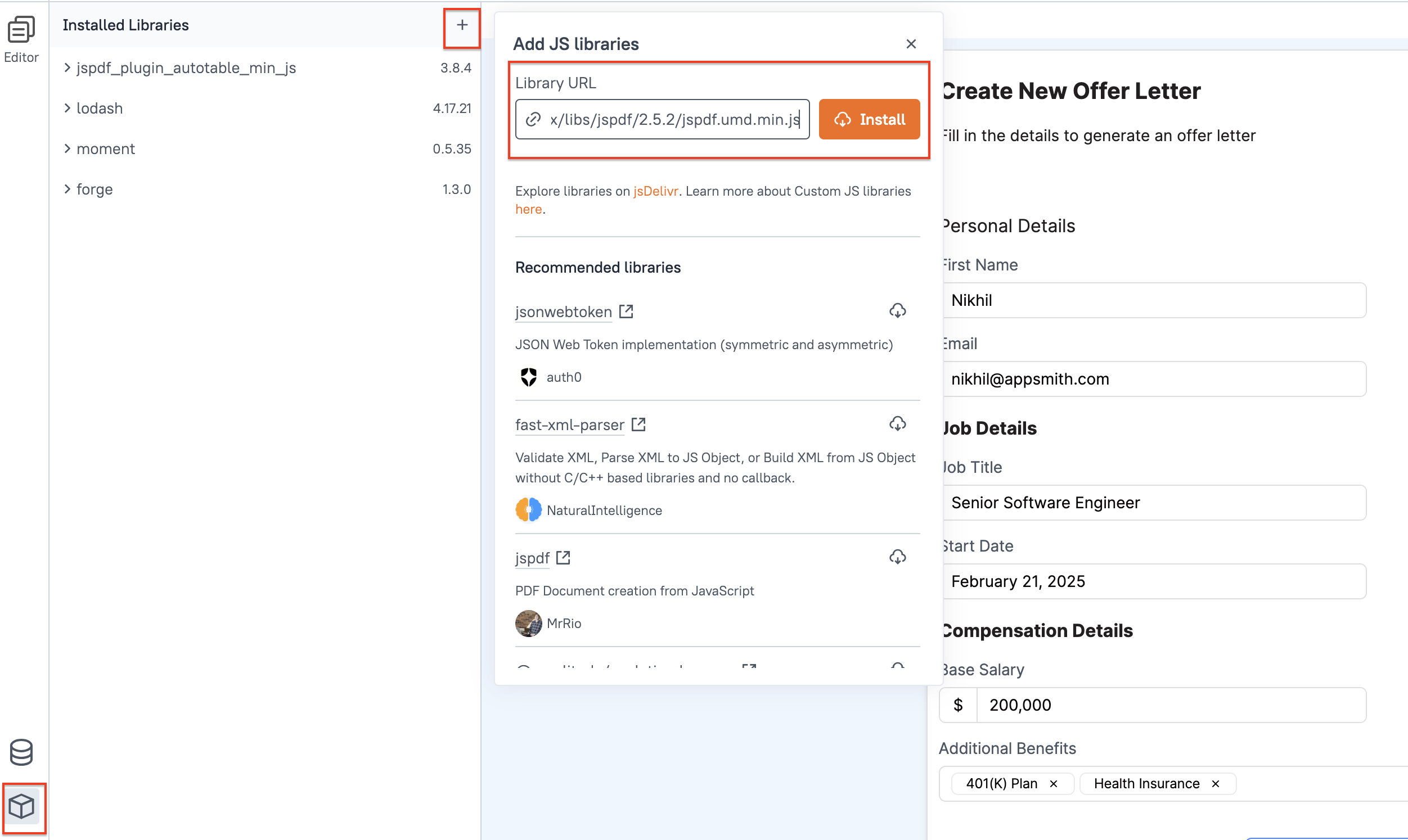
- Create a new JSObject from the JS tab to define the logic for generating the PDF using the JS library.
Example: If you want to generate a offer letter based on the inputs provided by a user, you can create a JSObject to handle the logic and formatting of the PDF.
export default {
// Data object to store dynamic values
data: {
date: moment().format("Do MMM, YYYY"),
firstName: firstName.text,
lastName: lastName.text,
email: email.text,
jobTitle: jobTitle.text,
salary: baseSalary.text,
},
// Function to generate the offer letter PDF
generateOfferPDF(data = this.data) {
// Initialize a new PDF document
const doc = new jspdf.jsPDF();
// Add the header
doc.text("Offer Letter", 10, 10);
doc.text(`Date: ${data.date}`, 10, 20);
// Add recipient details
doc.text(`To: ${data.firstName} ${data.lastName}`, 10, 30);
doc.text(`Email: ${data.email}`, 10, 40);
// Add the body of the offer letter
doc.text(`Dear ${data.firstName},`, 10, 60);
doc.text(`We are pleased to offer you the position of ${data.jobTitle}.`, 10, 70); // Job title information
doc.text(`Your annual salary will be ${data.salary}.`, 10, 80); // Salary details
// Add acceptance section
doc.text("If you accept this offer, please sign below:", 10, 100);
doc.text("Signature: __________________________", 10, 110); // Signature placeholder
doc.text("Date: __________________________", 10, 120);
// Return the PDF as a data URI string (can be used to preview/download the PDF)
return doc.output("datauristring");
},
};
- The code dynamically generates an offer letter PDF by retrieving user inputs (name, email, job title, salary) and formatting them using
jspdf.jsPDF()
. - The data object stores values from input fields, and the
generateOfferPDF
function uses these values to populate the PDF content. - The final PDF is returned as a data URI, ready to be displayed or downloaded.
- Drag a Document Viewer widget and set its Document link property to:
{{PDFUtils.generateOfferPDF()}}
This displays the offer letter PDF generated by the generateOfferPDF
function, allowing you to preview the offer letter based on the input values provided.
-
Connect to the SMTP datasource and create a new Send Email query. For more information, see How to Configure SMTP Datasource.
-
Configure the email query properties such as To, Subject, and Body. For the Attachment property, use the Base64-encoded PDF data.
Example:
{
"attachments": [
{
"type": "application/pdf",
"data": "{{PDFUtils.generateOfferPDF().replace('data:application/pdf;filename=generated.pdf;base64,', '')}}",
"name": "{{firstName.text}}.pdf",
"dataFormat": "Base64"
}
]
}
- Drag a Button widget and set its onClick event to run the Send Email query.
With this, you can dynamically generate a PDF based on your input and send it via email. You can also style your PDF as needed.
See Also:
- Template - Offer Letter Generation